Web Server with MicroPython
This program turns your Pico W into a small web server. The web page has two links on it. One link will turn the on-board LED on and the other link will turn the LED off.
Screen image of Pico W Web Server:
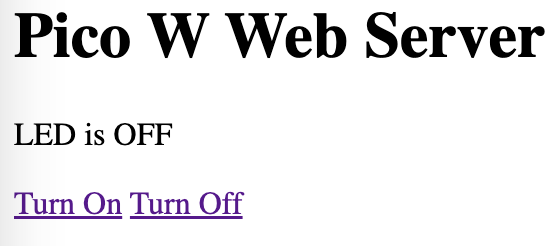
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88 | # Code taken from https://www.cnx-software.com/2022/07/03/getting-started-with-wifi-on-raspberry-pi-pico-w-board/
import network
import socket
import time
import secrets
from machine import Pin
# Select the onboard LED
led = machine.Pin("LED", machine.Pin.OUT)
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect(secrets.SSID, secrets.PASSWORD)
stateis = "LED is OFF"
html = """<!DOCTYPE html>
<html>
<head>
<title>Web Server On Pico W </title>
</head>
<body>
<h1>Pico Wireless Web Server</h1>
<p>%s</p>
<a href="/light/on">Turn On</a>
<a href="/light/off">Turn Off</a>
</body>
</html>
"""
# Wait for connect or fail
max_wait = 10
while max_wait > 0:
if wlan.status() < 0 or wlan.status() >= 3:
break
max_wait -= 1
print('waiting for connection...')
time.sleep(1)
# Handle connection error
if wlan.status() != 3:
raise RuntimeError('network connection failed')
else:
print('We are connected to WiFI access point:', secrets.SSID)
status = wlan.ifconfig()
print( 'The IP address of the pico W is:', status[0] )
# Open socket
addr = socket.getaddrinfo('0.0.0.0', 80)[0][-1]
print('addr:', addr)
s = socket.socket()
#if not addr:
s.bind(addr)
s.listen(1)
print('listening on', addr)
# Listen for connections
while True:
try:
cl, addr = s.accept()
print('client connected from', addr)
request = cl.recv(1024)
print(request)
request = str(request)
led_on = request.find('/light/on')
led_off = request.find('/light/off')
print( 'led on = ' + str(led_on))
print( 'led off = ' + str(led_off))
if led_on == 6:
print("led on")
led.value(1)
stateis = "LED is ON"
if led_off == 6:
print("led off")
led.value(0)
stateis = "LED is OFF"
# generate the we page with the stateis as a parameter
response = html % stateis
cl.send('HTTP/1.0 200 OK\r\nContent-type: text/html\r\n\r\n')
cl.send(response)
cl.close()
except OSError as e:
cl.close()
print('connection closed')
|
References